Tìm hiểu Dapper Micro ORM – một thư viện giúp tối ưu hóa truy vấn cơ sở dữ liệu trong ASP.NET Core Web API. Hướng dẫn cài đặt, cấu hình và sử dụng Dapper để tăng hiệu suất xử lý dữ liệu với các ví dụ chi tiết.
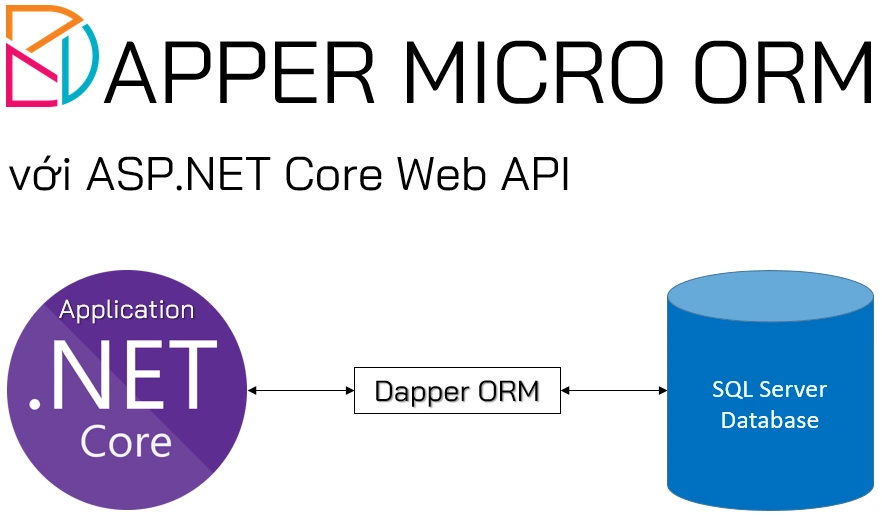
Giới thiệu về Dapper Micro ORM
Dapper là một Micro ORM (Object-Relational Mapper) nhẹ, được phát triển bởi StackExchange. Dapper giúp tăng hiệu suất truy vấn cơ sở dữ liệu trong .NET nhờ tối ưu hóa việc ánh xạ dữ liệu giữa các đối tượng C# và cơ sở dữ liệu. Khác với Entity Framework, Dapper không cung cấp cơ chế theo dõi thay đổi (Change Tracking) mà hoạt động dựa trên SQL thuần, giúp truy vấn nhanh hơn.
Lợi ích của Dapper
- Hiệu suất cao: Dapper nhanh hơn Entity Framework do không theo dõi trạng thái đối tượng.
- Dễ sử dụng: Cú pháp đơn giản, linh hoạt với SQL thuần.
- Hỗ trợ nhiều kiểu dữ liệu: Tương thích với các kiểu dữ liệu như
int
,string
,DateTime
,List<T>
,Dictionary<K,V>
,… - Tích hợp dễ dàng: Dapper có thể kết hợp với các ORM khác như Entity Framework hoặc NHibernate.
Nhược điểm của Dapper
- Không có tính năng Change Tracking: Cần viết SQL thủ công để cập nhật dữ liệu.
- Phụ thuộc vào SQL thuần: Dapper không hỗ trợ LINQ như Entity Framework.
Cài đặt Dapper Micro ORM trong ASP.NET Core Web API
Tạo dự án ASP.NET Core Web API
Mở Visual Studio 2019 hoặc Visual Studio 2022, tạo một dự án mới theo các bước sau:
- Chọn Create a new project → Chọn ASP.NET Core Web API.
- Đặt tên dự án, chọn .NET phiên bản mới nhất (ví dụ: .NET 6 hoặc .NET 7).
- Click Create.
Cài đặt Dapper qua NuGet
Mở Package Manager Console và chạy lệnh sau:
Install-Package Dapper
Hoặc sử dụng .NET CLI:
dotnet add package Dapper
Cấu hình Dapper Micro ORM trong ASP.NET Core
Thiết lập chuỗi kết nối
Mở appsettings.json và thêm thông tin kết nối:
{
"ConnectionStrings": {
"DefaultConnection": "Server=localhost;Database=TestDB;User Id=sa;Password=yourpassword;"
}
}
Tạo lớp DapperContext.cs
Tạo thư mục Data
và thêm tệp DapperContext.cs:
using System.Data;
using System.Data.SqlClient;
using Microsoft.Extensions.Configuration;
public class DapperContext
{
private readonly IConfiguration _configuration;
private readonly string _connectionString;
public DapperContext(IConfiguration configuration)
{
_configuration = configuration;
_connectionString = _configuration.GetConnectionString("DefaultConnection");
}
public IDbConnection CreateConnection() => new SqlConnection(_connectionString);
}
Thêm vào Program.cs (hoặc Startup.cs
nếu dùng .NET 5 trở xuống):
builder.Services.AddSingleton<DapperContext>();
Sử dụng Dapper Micro ORM trong Repository Pattern
Tạo Model Employee
Thêm thư mục Models và tạo lớp Employee.cs
:
public class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public string Position { get; set; }
public decimal Salary { get; set; }
}
Tạo Repository IEmployeeRepository.cs
Thêm thư mục Repositories và tạo Interface:
using System.Collections.Generic;
using System.Threading.Tasks;
public interface IEmployeeRepository
{
Task<IEnumerable<Employee>> GetEmployees();
Task<Employee> GetEmployeeById(int id);
Task<int> AddEmployee(Employee employee);
Task<int> UpdateEmployee(Employee employee);
Task<int> DeleteEmployee(int id);
}
Triển khai EmployeeRepository.cs
using Dapper;
using System.Collections.Generic;
using System.Data;
using System.Threading.Tasks;
public class EmployeeRepository : IEmployeeRepository
{
private readonly DapperContext _context;
public EmployeeRepository(DapperContext context)
{
_context = context;
}
public async Task<IEnumerable<Employee>> GetEmployees()
{
var query = "SELECT * FROM Employees";
using var connection = _context.CreateConnection();
return await connection.QueryAsync<Employee>(query);
}
public async Task<Employee> GetEmployeeById(int id)
{
var query = "SELECT * FROM Employees WHERE Id = @Id";
using var connection = _context.CreateConnection();
return await connection.QueryFirstOrDefaultAsync<Employee>(query, new { Id = id });
}
public async Task<int> AddEmployee(Employee employee)
{
var query = "INSERT INTO Employees (Name, Position, Salary) VALUES (@Name, @Position, @Salary)";
using var connection = _context.CreateConnection();
return await connection.ExecuteAsync(query, employee);
}
public async Task<int> UpdateEmployee(Employee employee)
{
var query = "UPDATE Employees SET Name = @Name, Position = @Position, Salary = @Salary WHERE Id = @Id";
using var connection = _context.CreateConnection();
return await connection.ExecuteAsync(query, employee);
}
public async Task<int> DeleteEmployee(int id)
{
var query = "DELETE FROM Employees WHERE Id = @Id";
using var connection = _context.CreateConnection();
return await connection.ExecuteAsync(query, new { Id = id });
}
}
Đăng ký Repository trong Program.cs:
builder.Services.AddScoped<IEmployeeRepository, EmployeeRepository>();
Tạo API Controller sử dụng Dapper Micro ORM
Tạo EmployeesController.cs
Thêm thư mục Controllers và tạo Controller:
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using System.Threading.Tasks;
[Route("api/[controller]")]
[ApiController]
public class EmployeesController : ControllerBase
{
private readonly IEmployeeRepository _repository;
public EmployeesController(IEmployeeRepository repository)
{
_repository = repository;
}
[HttpGet]
public async Task<IActionResult> GetEmployees()
{
var employees = await _repository.GetEmployees();
return Ok(employees);
}
[HttpGet("{id}")]
public async Task<IActionResult> GetEmployeeById(int id)
{
var employee = await _repository.GetEmployeeById(id);
if (employee == null) return NotFound();
return Ok(employee);
}
[HttpPost]
public async Task<IActionResult> AddEmployee(Employee employee)
{
await _repository.AddEmployee(employee);
return CreatedAtAction(nameof(GetEmployeeById), new { id = employee.Id }, employee);
}
[HttpPut("{id}")]
public async Task<IActionResult> UpdateEmployee(int id, Employee employee)
{
if (id != employee.Id) return BadRequest();
await _repository.UpdateEmployee(employee);
return NoContent();
}
[HttpDelete("{id}")]
public async Task<IActionResult> DeleteEmployee(int id)
{
await _repository.DeleteEmployee(id);
return NoContent();
}
}
Kết luận
Dapper là một lựa chọn tuyệt vời cho những ai muốn hiệu suất cao và dễ kiểm soát truy vấn SQL trong ASP.NET Core Web API. So với Entity Framework, Dapper Micro ORM đơn giản hơn, ít tiêu tốn tài nguyên và thích hợp với các ứng dụng cần tối ưu hóa tốc độ xử lý dữ liệu.
Bạn có thể kết hợp Dapper Micro ORM với Stored Procedures, Transaction và Async Programming để tăng hiệu suất xử lý dữ liệu. Nếu bạn đang tìm kiếm một ORM nhẹ, linh hoạt và dễ sử dụng, Dapper là một giải pháp đáng cân nhắc.
CÔNG TY TNHH GIẢI PHÁP CÔNG NGHỆ TRANG DESIGNER
Trang Designer chuyên thiết kế website chuẩn SEO, thiết kế logo toàn diện giúp doanh nghiệp xây dựng một thương hiệu mạnh và bán hàng hiệu quả trên các nền tảng số cho nhiều lĩnh vực kinh doanh.
Vui lòng liên hệ: 138 Hiền Vương, Phường Phú Thạnh, Quận Tân Phú, TP. Hồ Chí Minh
Điện thoại: 0903.728.335
Website: www.trangdesigner.id.vn